One of the powerful functionalities that Joomla offers is XML-RPC (XML Remote Procedure Call). This protocol enables communication between a client and a server over HTTP, making it possible to call methods and exchange data. In this article, we will explore what XML-RPC is, how to implement it in Joomla using PHP, and best practices for using XML-RPC in your applications.
What is XML-RPC?
XML-RPC is a remote procedure call (RPC) protocol that uses XML to encode its calls and HTTP as a transport mechanism. It allows remote systems to communicate with each other by invoking methods and passing parameters over the internet. The protocol is simple, lightweight, and language-agnostic, making it suitable for various applications.
Key Features of XML-RPC
- Simplicity: XML-RPC is easy to implement and understand, with straightforward data types and structures.
- Language Agnostic: It can be used with any programming language that can parse XML and send HTTP requests.
- Extensibility: XML-RPC can be extended to include additional functionalities and custom methods.
Setting Up XML-RPC in Joomla
To use XML-RPC in Joomla, you’ll need to follow a series of steps to configure your Joomla environment and create a PHP client that communicates with it.
Step 1: Enabling XML-RPC in Joomla
By default, Joomla may not have XML-RPC enabled. To ensure it is active, follow these steps:
- Log in to the Joomla Administrator Panel: Go to
http://yourdomain.com/administrator
and enter your credentials. - Access Global Configuration: Navigate to System > Global Configuration.
- Server Tab: Click on the Server tab.
- Enable XML-RPC: Look for the option labeled Enable XML-RPC and set it to Yes.
- Save Settings: Click Save & Close to apply the changes.
Step 2: Creating a Custom XML-RPC Service
To create a custom XML-RPC service in Joomla, you’ll need to define the functions that can be called remotely.
- Create a New PHP File: In your Joomla installation directory, navigate to the
components
folder. Create a new folder namedmyxmlrpc
and create a file namedmyxmlrpc.php
inside it. - Define the XML-RPC Functions: In
myxmlrpc.php
, start by including the necessary Joomla framework files:defined('_JEXEC') or die; // Load Joomla framework require_once JPATH_BASE . '/includes/defines.php'; require_once JPATH_BASE . '/includes/framework.php'; // Initialize Joomla application $app = JFactory::getApplication('site');
- Implement XML-RPC Functions: Below the initialization code, define your XML-RPC functions. For example, let’s create a function to get the current site title.
function getSiteTitle() { return JFactory::getConfig()->get('sitename'); }
- Register the Methods: You will need to use a library that handles XML-RPC requests. Joomla includes the necessary classes, so you can register your methods as follows:
// Include XML-RPC library require_once JPATH_LIBRARIES . '/joomla/rpc/rpc.php'; // Create an XML-RPC server $server = new JRPCServer(); // Register your method $server->registerMethod('getSiteTitle', 'getSiteTitle'); // Handle the request $server->service();
- Save Your PHP File: Ensure that all your code is correct and save the
myxmlrpc.php
file.
Step 3: Creating an XML-RPC Client in PHP
Now that you have your XML-RPC service set up in Joomla, you can create a PHP client to interact with it.
- Create a New PHP Client File: Create a new file named
client.php
in your local development environment. - Include XML-RPC Library: Use the built-in PHP XML-RPC library or a third-party library like
Zend_XmlRpc
. For this example, we will use PHP’s built-in functionality. - Write the Client Code: Here’s how you can create a simple PHP client that calls the
getSiteTitle
method on your Joomla XML-RPC service:<?php // Define the URL of the XML-RPC service $url = 'http://yourdomain.com/components/myxmlrpc/myxmlrpc.php'; // Prepare the XML-RPC request $request = xmlrpc_encode_request('getSiteTitle'); // Send the request $response = file_get_contents($url, false, stream_context_create([ 'http' => [ 'method' => 'POST', 'header' => 'Content-Type: text/xml', 'content' => $request, ], ])); // Decode the response $result = xmlrpc_decode($response); // Check for errors if (xmlrpc_is_fault($result)) { echo "Error: {$result['faultString']}"; } else { echo "Site Title: $result"; } ?>
- Run the Client: Open
client.php
in your browser to see the result. If everything is configured correctly, you should see the site title returned from your Joomla installation.
Step 4: Testing Your XML-RPC Setup
- Check for Errors: Make sure to check the Joomla error logs if you encounter any issues.
- Verify Network Connectivity: Ensure that your client can reach the Joomla server over HTTP.
Step 5: Securing Your XML-RPC Service
Security is critical when exposing services over XML-RPC. Here are some best practices to secure your XML-RPC implementation:
- Authentication: Implement authentication mechanisms to ensure that only authorized users can access your XML-RPC methods.
- Input Validation: Sanitize and validate all incoming data to prevent injection attacks.
- Limit Methods: Only expose the methods that are necessary for your application.
- Use HTTPS: Always use HTTPS to encrypt the data transmitted between the client and server.
Step 6: Exploring Advanced Features
Once you have a basic XML-RPC implementation, you can explore advanced features like:
- Batch Requests: XML-RPC supports batch requests, allowing you to call multiple methods in a single request.
- Complex Data Types: You can work with complex data types, such as arrays and structs, to exchange more intricate data between your client and server.
- Error Handling: Implement robust error handling to manage exceptions and provide meaningful error messages.
Joomla xml-rpc for php, it’s dev feature!
Integrating XML-RPC in Joomla using PHP allows you to create powerful remote communications between your Joomla site and other applications. By following the steps outlined in this guide, you can set up XML-RPC services and clients, enabling you to extend your website’s functionality and improve user interactions.
From creating simple methods to implementing security measures, XML-RPC offers a straightforward way to make your Joomla site more dynamic. Whether you’re developing a custom application or integrating with third-party services, XML-RPC can enhance your Joomla experience significantly. Happy coding!
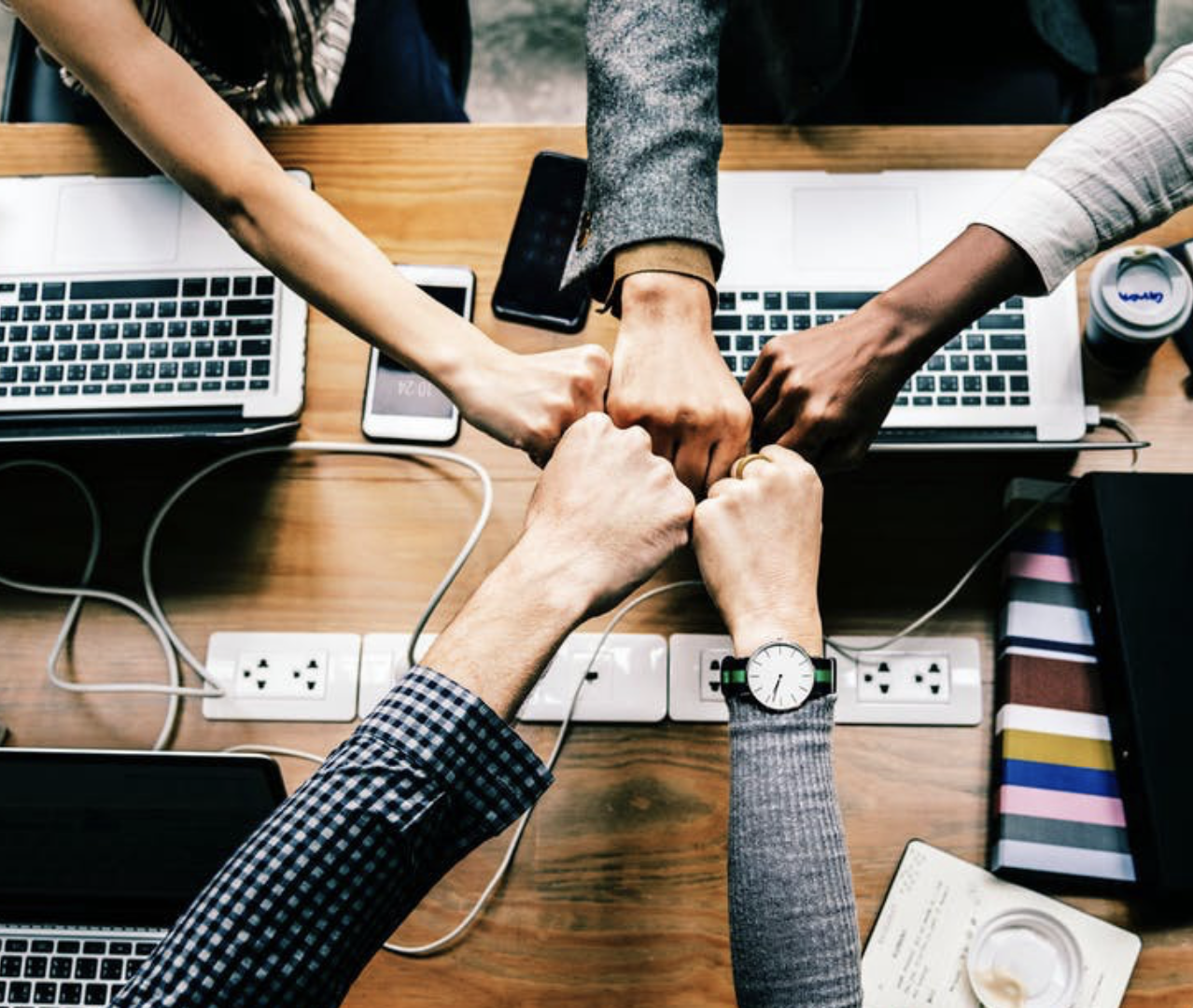
Hello everyone! We’re Galussothemes content team, we love to write new blogs for WordPress everyday! Galusso Content Team is a part of IT4GO – The company houses talented young people who hold a strong passion for website development, designing, and marketing.