The “Call to undefined method” error is a PHP error that occurs when you try to invoke a method on an object that does not have that method defined. This can happen for several reasons:
- Typographical Errors: A simple typo in the method name can lead to this error.
- Class Inheritance Issues: If the method is supposed to be inherited from a parent class but is missing, you will encounter this error.
- Namespace Issues: If you are using namespaces but have not properly imported the class, the method call may fail.
- Version Compatibility: The method may have been removed or changed in the version of Joomla you are using.
Common Scenarios Leading to the Error
1. Typographical Errors
One of the simplest causes of this error is a typo in the method name. PHP is case-sensitive, so a mismatch in capitalization can lead to a failure to find the method.
2. Class Inheritance Issues
If you are working with classes that extend other classes, make sure that the method you are trying to call is defined in the correct class. If the method is defined in a parent class but is not accessible due to visibility constraints (like being declared as private
), you will encounter this error.
3. Namespace Issues
PHP namespaces allow for better organization of code but can also introduce complexity. If you are using namespaced classes, ensure that you are importing the correct namespace at the top of your PHP file.
4. Version Compatibility
Joomla 4 has introduced various changes and deprecated certain methods that existed in earlier versions. If you are migrating from Joomla 3 to Joomla 4, you may encounter this issue if your code references methods that have been removed.
Troubleshooting “Call to Undefined Method” Errors
Step 1: Review the Error Message
When you encounter this error, the first step is to review the error message carefully. It usually provides information about which method is undefined and in which file the error occurred. For example:
Fatal error: Uncaught Error: Call to undefined method MyClass::myUndefinedMethod() in /path/to/file.php:10
Step 2: Check Your Code
- Locate the Method Call: Open the file mentioned in the error message and navigate to the line of code where the error occurs.
- Verify Method Name: Check the method name for any spelling errors or incorrect capitalization. Ensure that the method exists in the class you are trying to call it from.
- Check for Inheritance: If the method is meant to be inherited, verify that it is defined in the parent class and is accessible.
class ParentClass { public function myMethod() { // Method code } } class ChildClass extends ParentClass { public function callParentMethod() { $this->myMethod(); // Ensure this method exists in ParentClass } }
Step 3: Verify Class Instantiation
Ensure that you are instantiating the correct class. If you have multiple classes with similar names, you may inadvertently be calling a method from the wrong class.
$object = new CorrectClass(); // Ensure this is the correct class
$object->myMethod();
Step 4: Check Namespaces
If you are using namespaces, ensure that you are referencing the class properly. Import the class at the top of your PHP file:
use Vendor\Package\MyClass;
$object = new MyClass();
$object->myMethod();
Step 5: Review Joomla Documentation
If you are migrating from Joomla 3 to Joomla 4, consult the Joomla 4 documentation to check for any changes in method names or classes. The official documentation often highlights deprecated methods and their replacements.
Step 6: Debugging
If you are still unable to resolve the issue, consider adding debugging statements to your code. Use var_dump()
or print_r()
to inspect the object before the method call, ensuring it is an instance of the expected class.
var_dump($object);
$object->myMethod(); // Check the object before calling the method
Best Practices to Avoid “Call to Undefined Method” Errors
1. Follow Naming Conventions
Adhere to consistent naming conventions for your methods and classes. This will help reduce typographical errors and make your code easier to read.
2. Use IDE Features
Utilize IDE features to check for method existence and auto-complete functionality. Modern IDEs can help you catch errors before runtime.
3. Document Your Code
Ensure that you document your methods clearly, specifying which class they belong to and any dependencies they may have. This will help you and other developers understand the code quickly.
4. Regularly Update Joomla
Keep your Joomla installation up to date. Regular updates often include fixes for known issues and improvements to the core codebase.
5. Use Composer
If you’re developing extensions, consider using Composer for dependency management. Composer helps manage libraries and their versions, making it easier to avoid compatibility issues.
So, Joomla 4 call to undefined method, it’s error from a component
The “Call to undefined method” error in Joomla 4 can be frustrating, but with careful troubleshooting and a systematic approach, you can quickly resolve it. By understanding the common causes of this error and following best practices, you can minimize the likelihood of encountering it in your development work.
Whether you are a seasoned Joomla developer or just starting, this guide provides the tools you need to identify and fix method-related issues effectively. Remember to leverage the Joomla documentation and community resources for additional support, and happy coding!
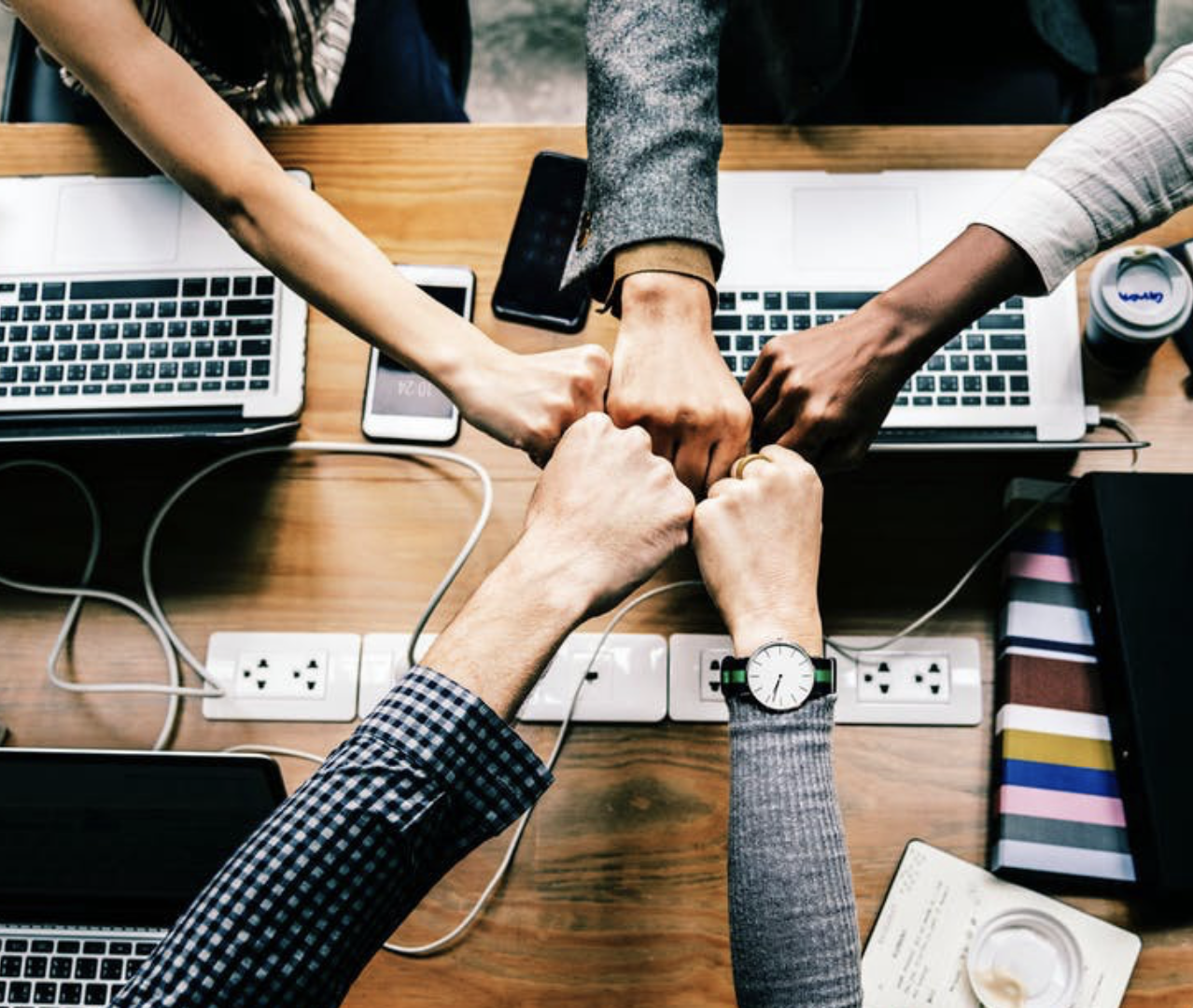
Hello everyone! We’re Galussothemes content team, we love to write new blogs for WordPress everyday! Galusso Content Team is a part of IT4GO – The company houses talented young people who hold a strong passion for website development, designing, and marketing.